In the world of Java programming, constructors play a vital role in initializing objects. A constructor in Java is a special method that is called when an object is instantiated. It has the same name as the class and is used to set initial values or execute startup logic for the object.This article delves deep into Java constructors, their types, and how they differ from methods.
What Is a Constructor in Java?
A constructor is a block of code used to initialize an object. Unlike regular methods, constructors do not have a return type—not even void
. They are automatically invoked when an object of the class is created. The primary function of a constructor is to assign values to the fields of the class or to perform any initialization process necessary for the class to function correctly.
Key Characteristics of Java Constructors
- Same Name as the Class: The name of the constructor must match the name of the class.
- No Return Type: Constructors do not have a return type, not even
void
. - Automatic Invocation: Called automatically when an object is created.
- Overloading Allowed: You can have multiple constructors in a class, differentiated by their parameter lists.
- Types of Constructors:
- Default Constructor: No parameters, provided by the compiler if no constructors are explicitly defined.
- Parameterized Constructor: Accepts arguments to initialize specific values.
Syntax of a Constructor
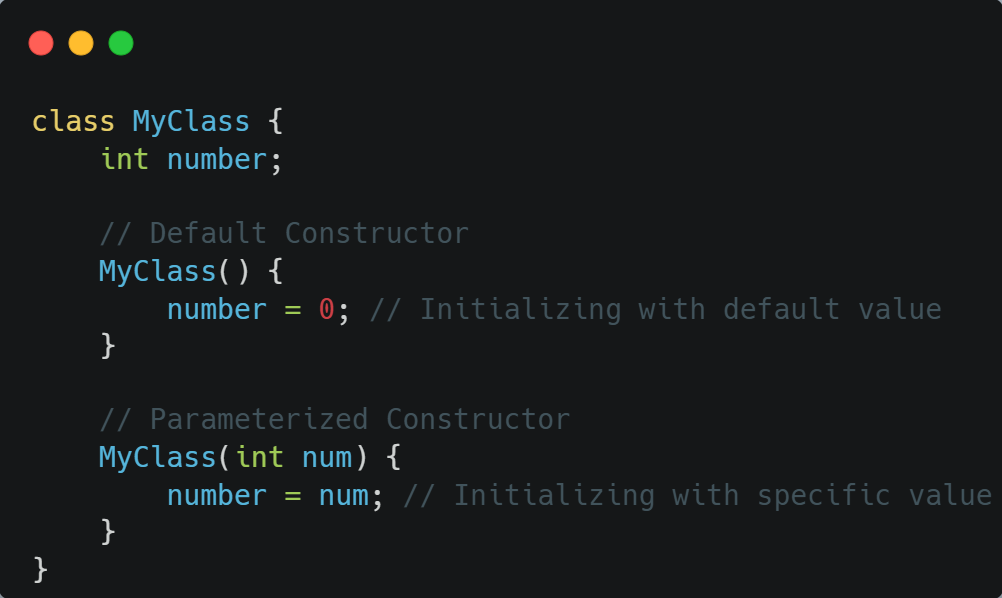
Types of Constructors in Java
1. Default Constructor
The default constructor is implicitly provided by Java if no constructors are defined in the class. It initializes object fields to their default values.
Example:
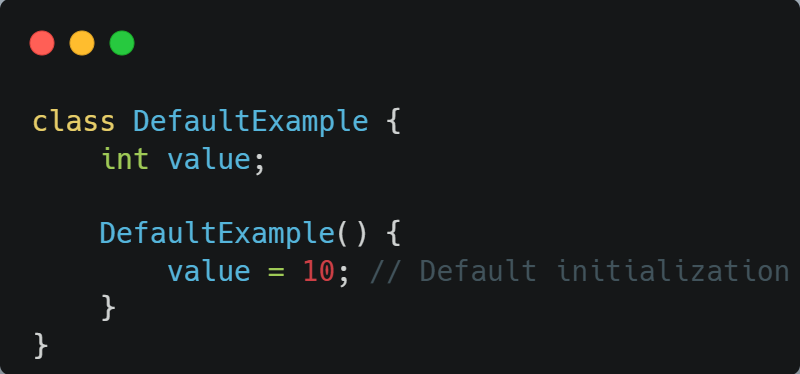
2. Parameterized Constructor
This constructor allows you to pass arguments to initialize the object fields with specific values.
Example:
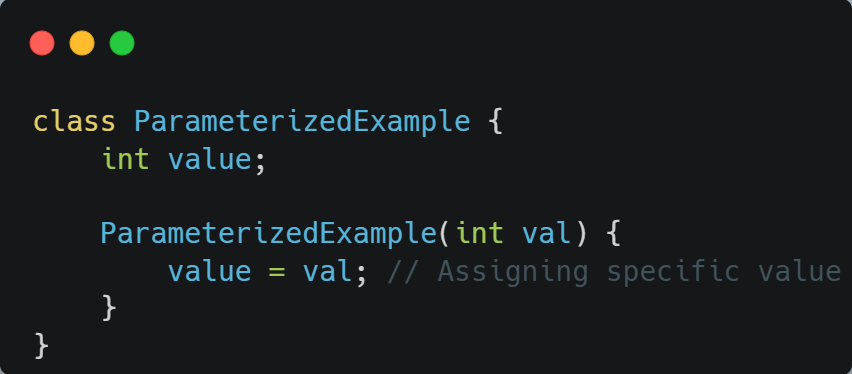
3. Copy Constructor
Although Java does not provide a built-in copy constructor, you can define one to copy the attributes of an existing object to a new object.
Example:
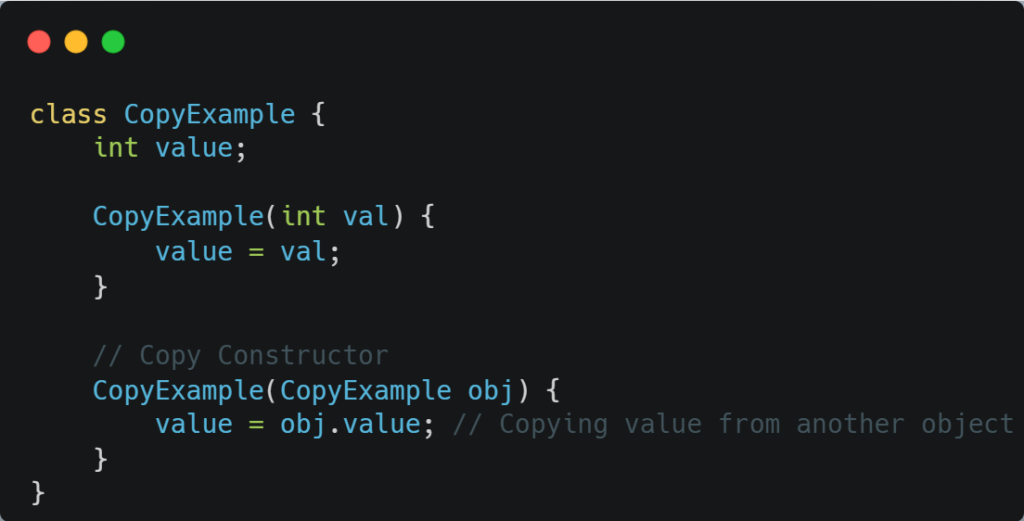
Difference Between Constructor and Method
While constructors and methods might appear similar, they serve distinct purposes. Here’s a detailed comparison:
Feature | Constructor | Method |
---|---|---|
Purpose | Initializes an object when it is created. | Performs a specific action or computation. |
Name | Must have the same name as the class. | Can have any name. |
Return Type | No return type, not even void . | Must have a return type or be void . |
Invocation | Automatically invoked during object creation. | Explicitly called using the object’s reference. |
Overloading | Supported. | Supported. |
Default Availability | Provided by Java if no constructor is defined. | No default methods are provided. |
How to Use Constructors Effectively
1. Constructor Overloading
Constructor overloading allows the creation of multiple constructors with different parameter lists, providing flexibility in object initialization.
Example:
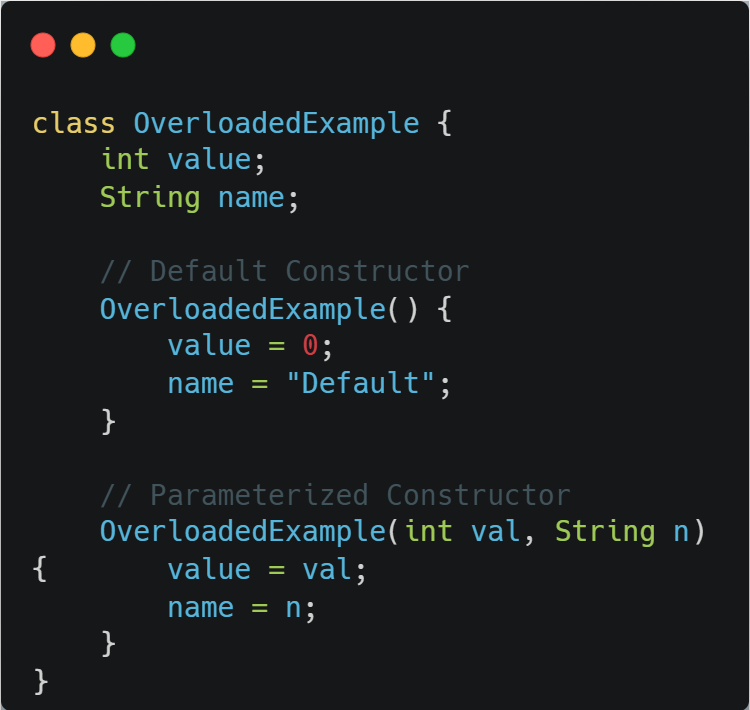
2. Chaining Constructors
Constructor chaining occurs when one constructor calls another constructor of the same class. Use the this()
keyword to achieve chaining.
Example:
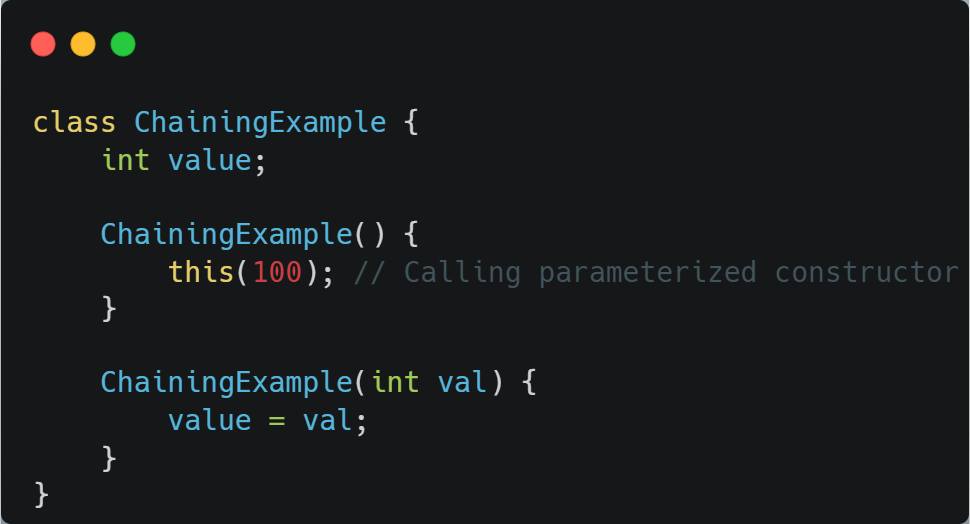
3. Use with Inheritance
In an inheritance hierarchy, the parent class’s constructor is called using the super()
keyword in the child class.
Example:
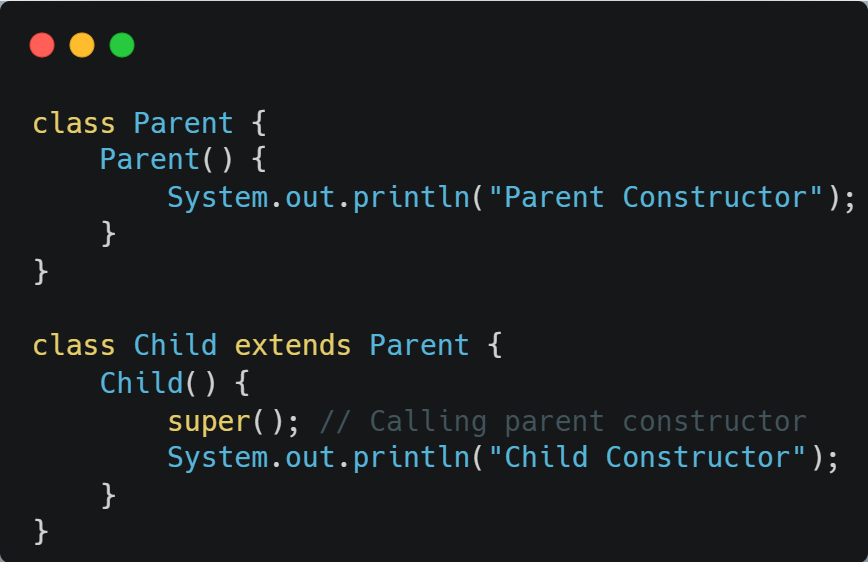
Advantages of Using Constructors
- Automatic Initialization: Simplifies the process of object initialization.
- Code Reusability: Constructor overloading enables different ways to initialize objects.
- Encapsulation: Ensures fields are initialized before the object is used.
Common Mistakes with Constructors
- Not Defining a Constructor: Relying solely on the default constructor may lead to unexpected behavior if fields need specific initialization.
- Infinite Recursion: Calling the same constructor within itself without proper termination logic can cause a
StackOverflowError
.
Conclusion
Understanding the concept of constructors in Java is fundamental for creating robust and efficient applications. They serve as the backbone for object initialization and provide a structured way to set up your program’s state. By mastering constructors and their applications, you can build cleaner and more maintainable codebases.
FAQs About Java Constructors
1. What is a constructor in Java?
A constructor in Java is a special method used to initialize objects. It is called automatically when an object is created and has the same name as the class.
2. How is a constructor different from a method in Java?
A constructor is used for initializing objects, while a method is used to perform specific actions. Constructors have no return type and are automatically invoked during object creation, whereas methods must be explicitly called and have a return type.
3. What are the types of constructors in Java?
There are three main types of constructors in Java:
- Default Constructor
- Parameterized Constructor
- Copy Constructor (user-defined)
4. Can a constructor be overloaded in Java?
Yes, constructors can be overloaded in Java by defining multiple constructors with different parameter lists.
5. What happens if no constructor is defined in a class?
If no constructor is defined, Java provides a default constructor that initializes the object with default values.
6. Can a constructor call another constructor in the same class?
Yes, this is called constructor chaining. It is done using the this()
keyword to call another constructor from the same class.
7. What is the role of the super()
keyword in constructors?
The super()
keyword is used in a child class constructor to call the parent class’s constructor, ensuring proper initialization in inheritance.