Introduction
In Java programming, variables are fundamental building blocks that store and manipulate data. Whether you’re a beginner or an experienced developer, understanding Java variables is essential for writing efficient and error-free code. In this tutorial, we will explore everything you need to know about Java variables, including their types, scope, initialization, and best practices.
By the end of this guide, you will have a solid understanding of how to use variables effectively in Java and avoid common pitfalls. Let’s dive in!
What is a Variable in Java?
A variable in Java is a container that holds data that can change during the execution of a program. It is a named memory location used to store values.
Syntax of Declaring a Variable in Java
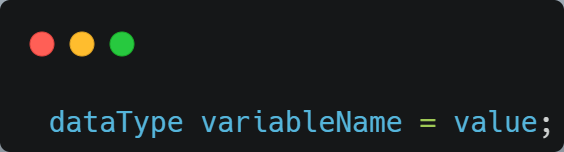
Example:
int age = 25;
Here, int
is the data type, age
is the variable name, and 25
is the assigned value.
Types of Variables in Java
Java provides different types of variables based on their usage and scope. These include:
1) Local Variables
Local variables are declared inside a method, constructor, or block and are accessible only within that scope.
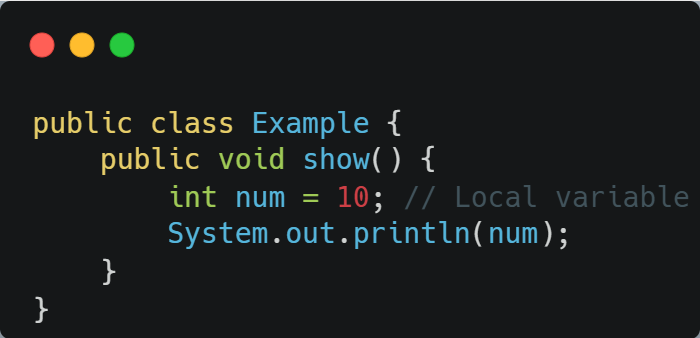
2)Instance Variables
Instance variables are declared inside a class but outside any method. They are associated with an object and are stored in the heap memory.

3)Static Variables
Static variables belong to the class rather than a specific object and are declared using the static
keyword.

Java Variable Data Types
Java is a strongly typed language, meaning each variable must have a declared type. Java’s data types fall into two categories:
1) Primitive Data Types
These include:
- int (Integer, 4 bytes) –
int num = 100;
- double (Floating point, 8 bytes) –
double price = 99.99;
- char (Character, 2 bytes) –
char grade = 'A';
- boolean (True/False, 1 bit) –
boolean isJavaFun = true;
2) Non-Primitive Data Types
These include:
- String –
String message = "Hello, Java!";
- Arrays –
int[] numbers = {1, 2, 3, 4};
- Objects –
Student student1 = new Student();
Variable Scope in Java
Understanding scope is crucial in Java programming. Variable scope determines where a variable can be accessed.
1) Method Scope
Variables declared inside a method are accessible only within that method.
2) Class Scope
Instance and static variables belong to a class and can be accessed by all methods within the class.
3) Block Scope
A variable inside {}
has limited accessibility.
if (true) {
int x = 10;
} // x is not accessible outside this block
Variable Initialization in Java
In Java, variables must be initialized before use.
1) Default Values of Variables
Java assigns default values to instance and static variables but not to local variables.
- int →
0
- double →
0.0
- boolean →
false
- String →
null
public class Example {
int num; // Defaults to 0
boolean flag; // Defaults to false
}
Constant Variable in Java
Java allows defining constant variable using the final
keyword. Constants cannot be changed once assigned.
final double PI = 3.14159;
Type Casting in Java
Java supports implicit and explicit type casting between data types.
1) Implicit Casting (Widening)
int num = 10;
double d = num; // Auto conversion
2) Explicit Casting (Narrowing)
double d = 10.5;
int num = (int) d; // Manual conversion
Best Practices for Using Variables in Java
To write efficient Java programs, follow these best practices:
- Use meaningful variable names (
age
instead ofa
) - Follow Java naming conventions (
camelCase
for variables) - Minimize the use of global variables
- Initialize variables before use
- Use constants for fixed values (
final
keyword)
Common Mistakes to Avoid
- Using uninitialized local variables
- Forgetting to assign values to final variables
- Using wrong data types (
int
instead ofdouble
for decimal values) - Overusing static variables (can lead to memory issues)
Conclusion
Understanding Java variables is a fundamental skill for any Java developer. From primitive types to object references, from local to static variables, Java provides a robust way to manage data efficiently. By following best practices and avoiding common pitfalls, you can write cleaner, more maintainable code.
Want to explore more Java concepts? Check out our Java Programming Tutorials.