Introduction
Java 8, introduced by Oracle in March 2014, is a revolutionary release in the Java ecosystem. It transformed the way we write, debug, and execute Java programs. Packed with modern programming constructs, Java 8 features brought improvements in performance, developer productivity, and support for functional programming paradigms. This article delves into the core features of Java 8, explains their concepts in a simple manner for Class 12 students, and illustrates their real-world applications.
Core Features of Java 8
- Lambda Expressions
- Stream API
- Optional Class
- Functional Interfaces
- Default and Static Methods in Interfaces
- Date and Time API
- Nashorn JavaScript Engine
- Parallel Arrays
- Type Annotations and Improved Compiler Warnings
Feature Explanation for Class 12 Students
1. Lambda Expressions
Lambda Expressions in Java 8 allow us to write concise code by simplifying the way functions are implemented. They eliminate the need for boilerplate code, making the syntax clean and functional.
Example:
Before Java 8:
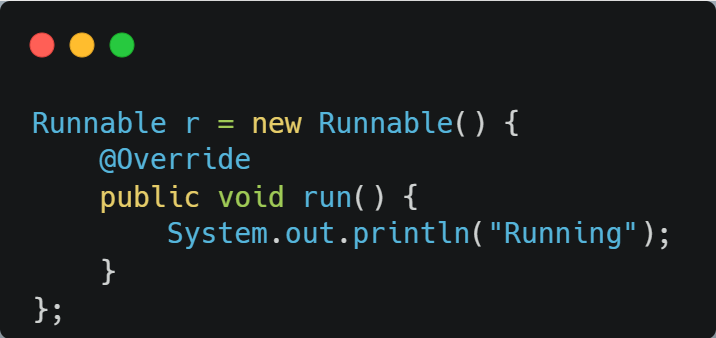
With Lambda:

2. Stream API
The Stream API helps in processing collections of data efficiently using functional-style operations. It supports operations like filtering, mapping, and reducing.
Example:

3. Optional Class
The Optional class is a container object used to handle null values safely. It helps prevent NullPointerExceptions.
Example:

4. Functional Interfaces
Functional interfaces are interfaces with a single abstract method. They can be implemented using Lambda Expressions.
Example:
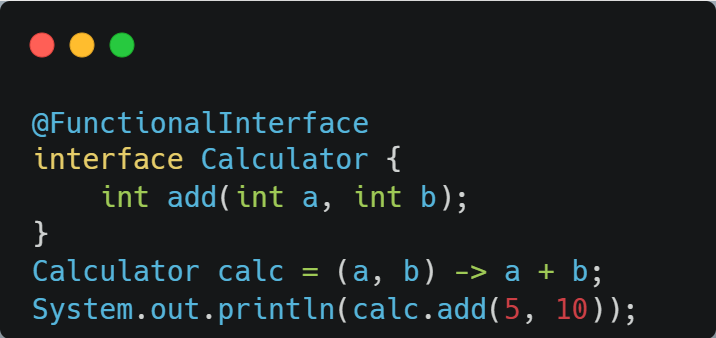
5. Default and Static Methods in Interfaces
Java 8 allows methods with implementations inside interfaces. This ensures backward compatibility.
Example:
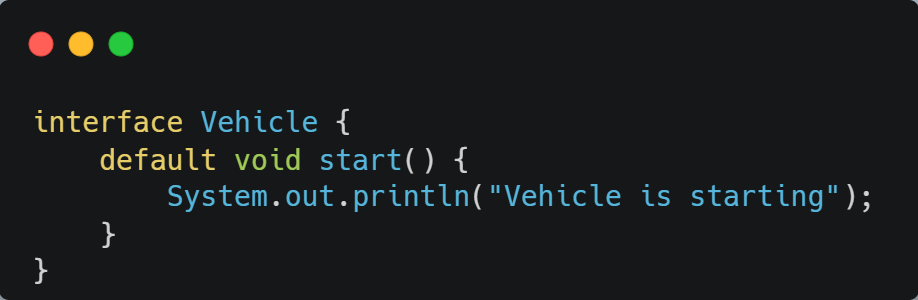
6. Date and Time API
The new Date and Time API solves the problems of the old java.util.Date
. It provides more flexibility and immutability.
Example:
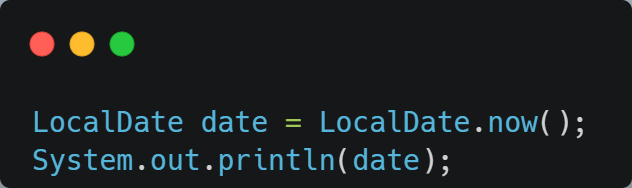
Implementation of Key Features
Implementing Lambda Expressions
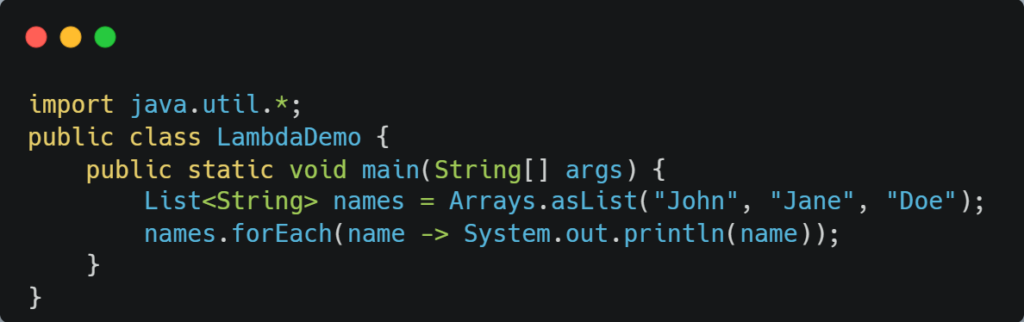
Using Stream API for Data Processing
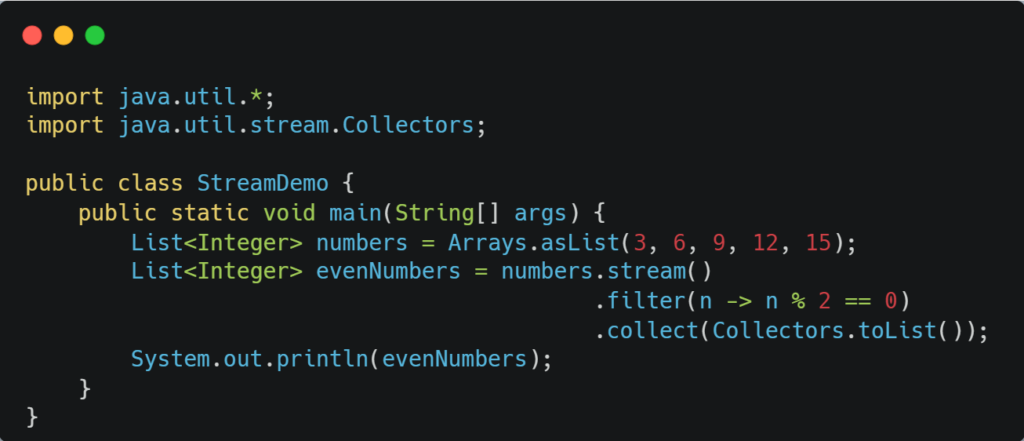
Use Cases of Java 8 Features
- Lambda Expressions: Useful in event handling, GUI programming, and scenarios involving anonymous inner classes.
- Stream API: Ideal for processing large datasets in data analytics and filtering logs in server applications.
- Optional Class: Enhances code robustness by preventing null-related bugs in enterprise applications.
- Date and Time API: Widely used in scheduling and time zone management for global applications.
- Default Methods in Interfaces: Simplifies extending functionalities of APIs without breaking existing implementations.
Advantages of Java 8 Features
- Improved Developer Productivity: Shorter and cleaner code with Lambda Expressions.
- Functional Programming: Adoption of modern programming paradigms.
- Better Performance: Stream API leverages multi-core processors for efficient data processing.
- Backward Compatibility: Default and static methods ensure older codebases remain compatible.
- Enhanced Date Handling: Solves common pitfalls of traditional date handling.
FAQ’s
Java 8 Interview Questions and Answers
Q1. What are the main features introduced in Java 8?
A:
- Lambda Expressions
- Stream API
- Functional Interfaces
- Default and Static Methods in Interfaces
- Optional Class
- New Date and Time API
- Nashorn JavaScript Engine
Q2. What is a Lambda Expression in Java 8?
A: Lambda Expression is a concise way to represent a function interface using an anonymous method. It enables functional programming and reduces boilerplate code.
Q3. Explain the purpose of the Stream API in Java 8.
A: The Stream API provides a way to process collections and other data sources in a functional style. It supports operations like filtering, mapping, and reducing, as well as parallel processing for better performance.
Q4. What is the Optional class, and how is it used?
A: The Optional
class helps handle null values safely by wrapping the value in a container. It prevents NullPointerException
.
Q5. What are functional interfaces? Can you name some examples?
A: A functional interface is an interface with exactly one abstract method, suitable for Lambda Expressions.
Examples:
Runnable
Callable
Comparator
Predicate
Function
Java 8 Interview Questions and Answers
Q1. What are the main features introduced in Java 8?
A:
- Lambda Expressions
- Stream API
- Functional Interfaces
- Default and Static Methods in Interfaces
- Optional Class
- New Date and Time API
- Nashorn JavaScript Engine
Q2. What is a Lambda Expression in Java 8?
A: Lambda Expression is a concise way to represent a function interface using an anonymous method. It enables functional programming and reduces boilerplate code.
Q3. Explain the purpose of the Stream API in Java 8.
A: The Stream API provides a way to process collections and other data sources in a functional style. It supports operations like filtering, mapping, and reducing, as well as parallel processing for better performance.
Q4. What is the Optional class, and how is it used?
A: The Optional
class helps handle null values safely by wrapping the value in a container. It prevents NullPointerException
.
Q5. What are functional interfaces? Can you name some examples?
A: A functional interface is an interface with exactly one abstract method, suitable for Lambda Expressions.
Examples:
Runnable
Callable
Comparator
Predicate
Function
Q6. What is the significance of default and static methods in interfaces?
A:
- Default methods allow methods with implementation in interfaces.
- Static methods provide utility methods that are specific to the interface.