Welcome to our Spring Framework blog series! Today, we’ll explore Dependency Injection (DI)—one of the core principles that makes Spring so powerful. DI simplifies object creation and reduces coupling between components, enabling more maintainable and testable code.
What is Dependency Injection?
Dependency Injection is a design pattern where the dependencies of an object are provided externally rather than being created within the object itself. This promotes flexibility and decoupling in your application.
Key Benefits of Dependency Injection
- Improved Testability: Mock objects can easily replace actual dependencies during testing.
- Reduced Boilerplate Code: Simplifies object creation by eliminating manual dependency management.
- Enhanced Flexibility: Makes it easier to switch between different implementations of a dependency.
- Loose Coupling: Ensures modules are independent of each other.
How Dependency Injection Works in Spring
In the Spring Framework, DI is implemented using the Inversion of Control (IoC) container. The IoC container manages the lifecycle of objects and their dependencies.
Steps Involved in Spring DI
- Define the dependencies in a configuration file (XML, Java-based, or annotations).
- The IoC container resolves these dependencies and injects them into the required objects.
Types of Dependency Injection in Spring
1. Constructor Injection
Dependencies are provided through the class constructor.
Example:
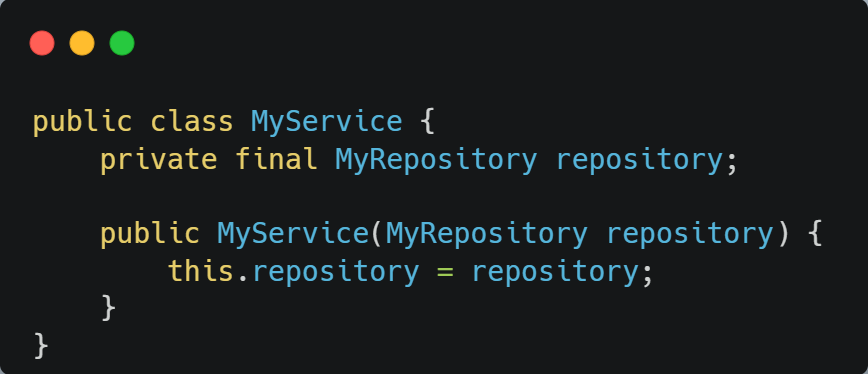
2. Setter Injection
Dependencies are provided using setter methods.
Example:
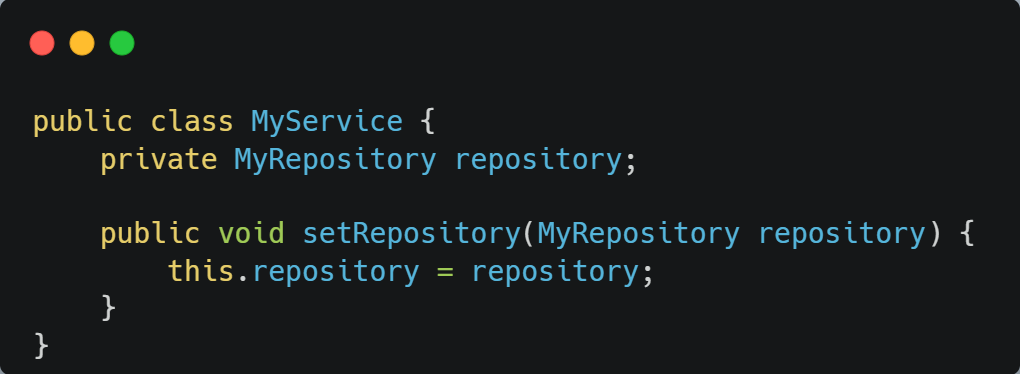
3. Field Injection
Dependencies are injected directly into fields using annotations like @Autowired
.
Example:
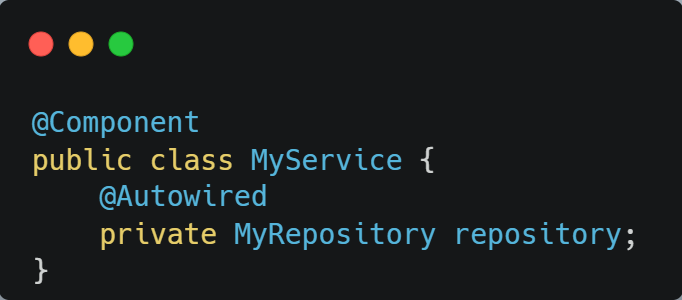
Best Practices for Using DI in Spring
- Prefer Constructor Injection: It ensures immutability and makes dependencies explicit.
- Minimize Field Injection: It’s harder to test and less transparent.
- Avoid Circular Dependencies: They can lead to runtime errors.
- Use Annotations Effectively: Spring annotations like
@Autowired
,@Component
, and@Qualifier
make DI seamless.
Real-World Example of Dependency Injection
Here’s a simple example of a Spring service layer using DI:
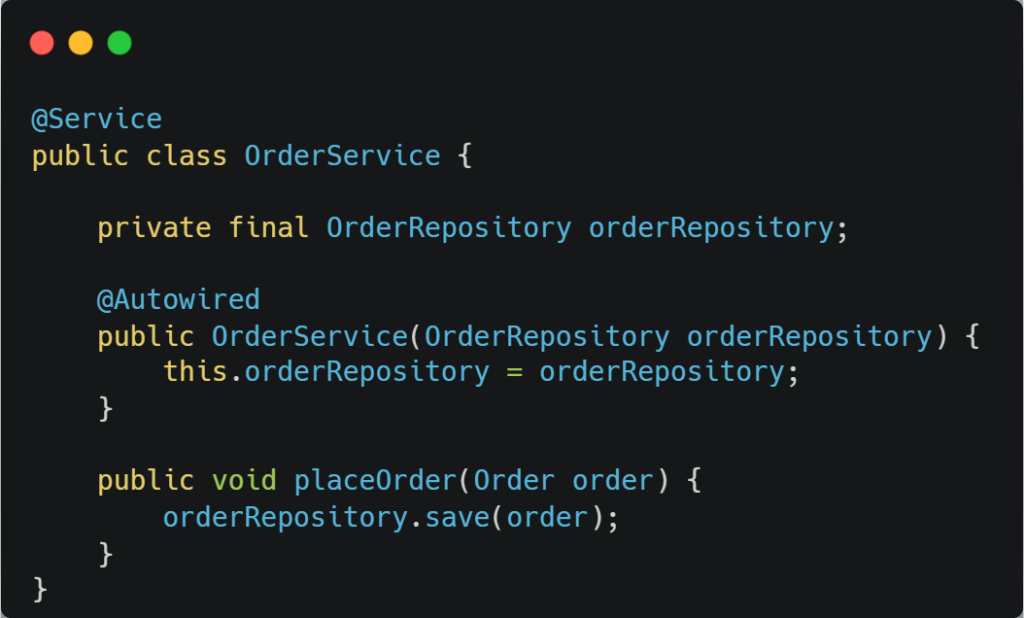
In this example, OrderService
depends on OrderRepository
. Spring’s IoC container automatically injects the dependency when the application starts.
Conclusion
Dependency Injection is the cornerstone of the Spring Framework. It simplifies application development by reducing coupling and improving code maintainability. By mastering DI, you’re on your way to building scalable and testable applications.We’ll explore 3. Inversion of Control (IoC) in greater depth, giving you more tools to create efficient applications.
FAQs about Dependency Injection in Spring
Q1. What is the main purpose of Dependency Injection?
DI decouples application components, making the code easier to manage and test.
Q2. Which type of DI is most preferred in Spring?
Constructor Injection is generally preferred for its clarity and immutability.
Q3. Can Dependency Injection work without annotations?
Yes, Spring supports XML and Java-based configurations for DI in addition to annotations.
Q4. What are common challenges with DI in Spring?
Circular dependencies and improper use of annotations can create runtime issues.