In the world of Java development, the Spring Framework stands as one of the most widely used platforms for building robust enterprise applications. At the core of Spring’s functionality is its Inversion of Control (IoC) container, which is primarily responsible for managing the lifecycle and configuration of application beans. One of the key components of the Spring IoC container is the ApplicationContext. This article delves into the role of the ApplicationContext, its features, benefits, and how it enhances Spring’s dependency injection mechanism.
What is ApplicationContext in Spring IoC?
The ApplicationContext is a powerful and advanced version of the BeanFactory in Spring. It is part of the Inversion of Control (IoC) container and serves as the central interface to interact with the Spring beans. The ApplicationContext offers a more feature-rich environment, providing capabilities like event propagation, bean post-processors, and internationalization support.
Unlike BeanFactory, the ApplicationContext is initialized eagerly, which means it loads all the beans at the startup of the application. This behavior helps in configuring and managing complex enterprise applications effectively.
Key Features of ApplicationContext
1. Bean Management
The ApplicationContext manages the lifecycle of beans, including their instantiation, dependency injection, initialization, and destruction. It ensures that beans are created and injected according to the configuration defined in either XML, annotations, or Java-based configuration.
2. Dependency Injection
Through the ApplicationContext, Spring’s dependency injection mechanism is seamlessly integrated. It allows developers to easily inject beans into one another, minimizing the need for explicit object creation and configuration, and promoting loose coupling between components.
3. Event Handling
The ApplicationContext provides event propagation, which is crucial for event-driven programming. With this feature, components can publish and listen to events in the Spring container. This allows for more flexible and decoupled communication between beans.
4. Support for AOP (Aspect-Oriented Programming)
ApplicationContext allows for easy integration of AOP into your Spring beans, enabling cross-cutting concerns such as logging, transaction management, and security to be applied in a declarative manner.
5. Internationalization (i18n)
Spring provides support for handling multiple languages and locales with the MessageSource interface, which is integrated into the ApplicationContext. This feature enables applications to be easily localized, enhancing the user experience across different regions.
6. Integration with Spring Web Modules
For web applications, the ApplicationContext integrates seamlessly with WebApplicationContext, which is a specialized context for web applications. This ensures proper management of beans in Spring MVC and related modules.
How ApplicationContext Works
The ApplicationContext works by reading configuration metadata that defines how beans should be created, injected, and initialized. This configuration can be defined in:
- XML Configuration: The traditional approach for bean definition.
- Annotation-Based Configuration: A more modern and concise approach using
@Configuration
and@Bean
. - Java-Based Configuration: Uses Java code to configure beans, offering type safety and flexibility.
Once the ApplicationContext is initialized, it provides access to all the beans defined within the configuration. Through dependency injection, these beans can be injected into other beans as needed.
Types of ApplicationContext
There are several implementations of the ApplicationContext that are suitable for different application needs:
1. ClassPathXmlApplicationContext
This implementation loads the context from an XML configuration file located in the classpath. It’s one of the most widely used methods for Spring bean configuration.
2. AnnotationConfigApplicationContext
This is used for Java-based configuration, providing a more modern approach to configuring Spring beans. It simplifies the process of bean registration by using annotations.
3. GenericWebApplicationContext
A variant of ApplicationContext for Spring Web MVC applications, allowing for the configuration and management of beans in web environments.
4. GenericWebApplicationContext (used in Spring Boot)
In Spring Boot, GenericWebApplicationContext is frequently used, especially for setting up web applications that are conventionally configured with @SpringBootApplication
.
ApplicationContext vs BeanFactory: Key Differences
While both BeanFactory and ApplicationContext are IoC containers in Spring, there are some notable differences between them:
Feature | BeanFactory | ApplicationContext |
---|---|---|
Initialization | Lazy initialization | Eager initialization (loads beans at startup) |
Features | Basic IoC container | Full-fledged IoC container with additional features like event propagation, AOP, and internationalization |
Event Handling | Not supported | Supported |
Internationalization | Not supported | Supports internationalization (i18n) |
AOP Support | Not supported | Supports AOP integration |
Web Support | No | Specialized support for web applications |
Suitable for | Simple applications or minimal configurations | Enterprise applications, web apps, and more complex environments |
Setting Up ApplicationContext
Setting up an ApplicationContext in Spring can be done in several ways. Below are two common methods:
1. XML-Based Configuration
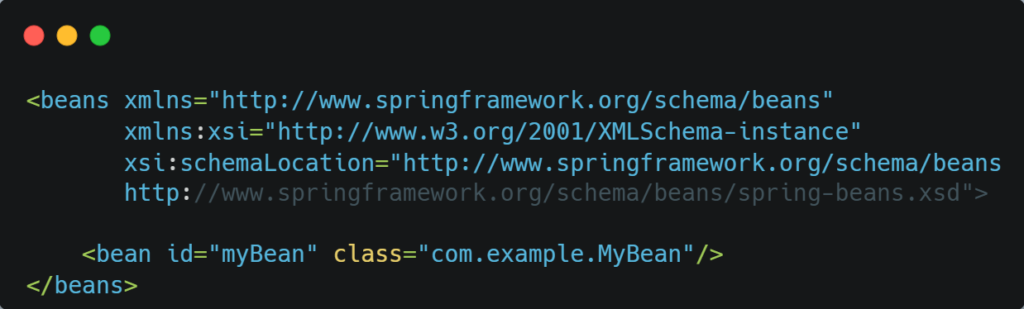
2.Annotation-Based Configuration
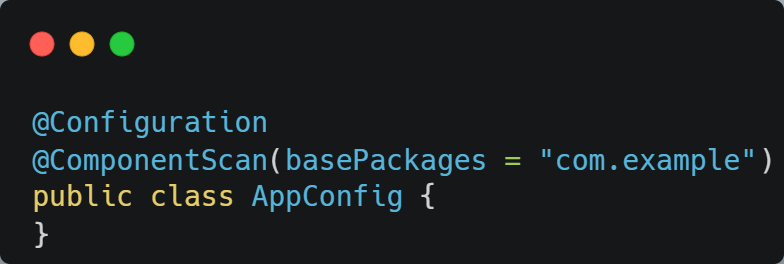
3.Java-Based Configuration
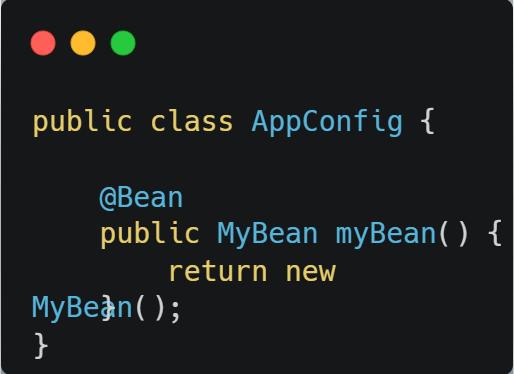
Best Practices for Using ApplicationContext
- Use ApplicationContext for Complex Applications
The ApplicationContext is ideal for complex enterprise applications where you need advanced features like event handling, AOP, and internationalization. - Avoid Circular Dependencies
While the ApplicationContext manages dependencies effectively, circular dependencies can still create problems. Ensure proper design to avoid such issues. - Lazy Initialization for Large Applications
For larger applications, consider using lazy initialization to optimize the startup time of your application. This ensures beans are created only when needed. - Use Profiles for Different Environments
With Spring’s @Profile annotation, you can specify different configurations for development, testing, and production environments, making your application more flexible and maintainable.
Conclusion
The ApplicationContext is a vital component in the Spring IoC container, providing essential features for enterprise-level applications. By leveraging the ApplicationContext, developers can manage beans efficiently, handle events, integrate AOP, and support internationalization. Its rich feature set makes it the go-to choice for building scalable and maintainable Java applications.